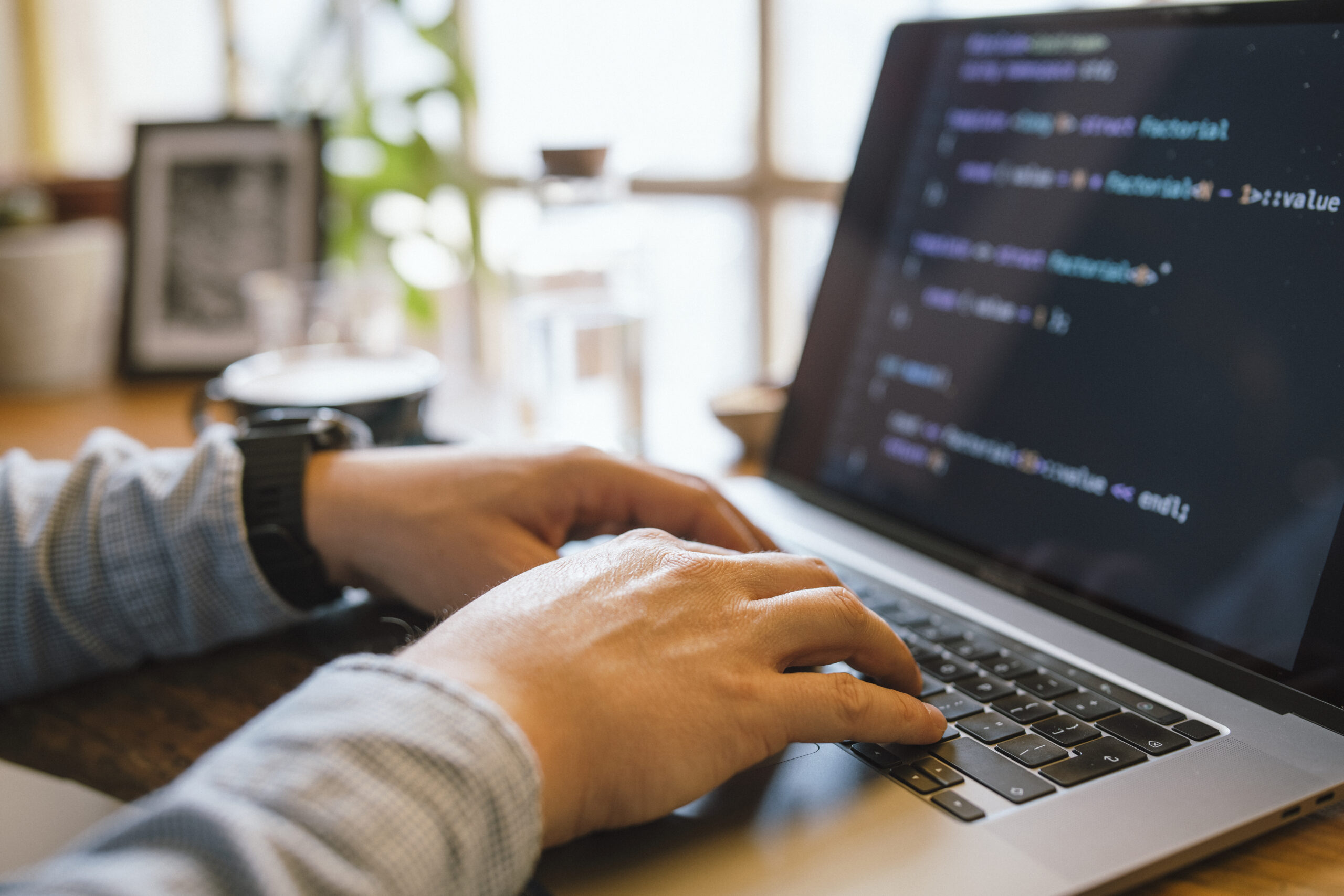
Debugging is Just about the most critical — nonetheless frequently disregarded — techniques in a very developer’s toolkit. It isn't really just about repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to think methodically to resolve troubles proficiently. No matter if you are a rookie or possibly a seasoned developer, sharpening your debugging skills can save several hours of irritation and radically help your efficiency. Listed here are several strategies that will help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the resources they use on a daily basis. Even though creating code is 1 Element of progress, being aware of tips on how to communicate with it successfully during execution is Similarly crucial. Present day progress environments arrive equipped with highly effective debugging capabilities — but lots of developers only scratch the surface of what these tools can perform.
Consider, such as, an Built-in Development Natural environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code on the fly. When utilised properly, they Permit you to observe accurately how your code behaves in the course of execution, which can be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish developers. They help you inspect the DOM, keep track of community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable duties.
For backend or procedure-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory administration. Discovering these resources could have a steeper Mastering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation control methods like Git to grasp code history, locate the exact second bugs ended up released, and isolate problematic variations.
Ultimately, mastering your equipment suggests likely outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your advancement setting making sure that when difficulties occur, you’re not missing in the dead of night. The greater you realize your resources, the more time you can spend solving the particular challenge instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently ignored — steps in effective debugging is reproducing the condition. Right before leaping to the code or creating guesses, developers require to produce a reliable setting or situation exactly where the bug reliably seems. Without the need of reproducibility, correcting a bug turns into a sport of prospect, generally resulting in wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you've got, the easier it gets to isolate the exact ailments below which the bug takes place.
When you’ve collected plenty of details, seek to recreate the trouble in your neighborhood surroundings. This may signify inputting exactly the same details, simulating comparable person interactions, or mimicking system states. If The problem seems intermittently, think about writing automated checks that replicate the edge situations or point out transitions involved. These exams not only support expose the condition but additionally protect against regressions in the future.
At times, The problem could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than distinct configurations. Applying tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the challenge isn’t merely a step — it’s a frame of mind. It necessitates tolerance, observation, along with a methodical method. But as soon as you can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible situation, You need to use your debugging tools much more successfully, check prospective fixes securely, and talk a lot more Obviously with the staff or people. It turns an summary grievance into a concrete challenge — and that’s where developers thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Erroneous. In lieu of viewing them as aggravating interruptions, developers should master to take care of error messages as direct communications in the system. They normally inform you what exactly occurred, where it happened, and in some cases even why it took place — if you understand how to interpret them.
Start by examining the concept cautiously As well as in comprehensive. A lot of developers, specially when beneath time pressure, look at the initial line and immediately get started generating assumptions. But deeper from the error stack or logs may perhaps lie the genuine root trigger. Don’t just duplicate and paste error messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Is it a syntax mistake, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can manual your investigation and place you toward the dependable code.
It’s also helpful to grasp the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java typically follow predictable patterns, and Mastering to recognize these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in All those cases, it’s vital to look at the context wherein the error occurred. Check out associated log entries, input values, and up to date variations within the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger concerns and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns a lot quicker, reduce debugging time, and become a much more productive and assured developer.
Use Logging Correctly
Logging is Among the most highly effective applications within a developer’s debugging toolkit. When employed properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood without having to pause execution or move in the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging amounts include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, INFO for typical situations (like prosperous start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for real issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure crucial messages and decelerate your process. Give attention to important situations, condition modifications, enter/output values, and demanding selection points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it requires to identify issues, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To properly detect and resolve bugs, builders need to tactic the procedure like a detective solving a mystery. This frame of mind can help stop working complex problems into workable sections and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the situation: error messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, gather as much pertinent details as you'll be able to with no leaping to conclusions. Use logs, exam scenarios, and person stories to piece jointly a clear image of what’s happening.
Subsequent, form hypotheses. Ask oneself: What could possibly be creating this behavior? Have any changes a short while ago been built to your codebase? Has this situation transpired prior to underneath related conditions? The target should be to slender down alternatives and detect probable culprits.
Then, examination your theories systematically. Make an effort to recreate the situation in the controlled environment. For those who suspect a selected operate or element, isolate it and verify if The problem persists. Like a detective conducting interviews, ask your code thoughts and Permit the outcomes guide you nearer to the truth.
Pay back near interest to compact aspects. Bugs frequently disguise while in the least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race issue. Be extensive and affected person, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may well hide the true issue, just for it to resurface later on.
Last of all, preserve notes on Anything you attempted and acquired. Just as detectives log their investigations, documenting your debugging course of action can conserve time for upcoming problems and enable Other folks have an understanding of your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique problems methodically, and grow to be simpler at uncovering concealed issues in advanced units.
Create Exams
Producing checks is one of the most effective approaches to transform your debugging competencies and General advancement performance. Checks not only assist catch bugs early but in addition function a security Internet that provides you self esteem when earning changes to the codebase. A properly-examined software is simpler to debug as it means that you can pinpoint particularly where by and when a dilemma takes place.
Get started with device tests, which focus on individual functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as predicted. Any time a exam fails, you promptly know wherever to seem, drastically lowering time spent debugging. Device assessments are Specially beneficial for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Upcoming, integrate integration tests and end-to-conclusion assessments into your workflow. These assist ensure that many parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate techniques with multiple parts or solutions interacting. If a little something breaks, your checks can inform you which part of the pipeline unsuccessful and below what ailments.
Composing checks also forces you to Imagine critically about your code. To check a characteristic properly, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug might be a powerful initial step. When the test fails constantly, you could give attention to repairing the bug and watch your check move when The difficulty is resolved. This technique ensures that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, speedier and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease disappointment, and sometimes see The problem from a new perspective.
When you're as well close to the code for as well very long, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code that you just wrote just hrs previously. On this state, your brain becomes fewer economical at issue-solving. A brief stroll, a coffee break, or even switching to another Developers blog undertaking for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of an issue once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, Specially in the course of lengthier debugging classes. Sitting down in front of a display screen, mentally caught, is not merely unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It gives your Mind House to breathe, improves your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing worthwhile when you take the time to reflect and evaluate what went Improper.
Start off by inquiring your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The answers often expose blind places in the workflow or knowing and enable you to Create more powerful coding behavior relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring issues or common issues—you can proactively prevent.
In crew environments, sharing Everything you've learned from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, supporting Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the very best builders aren't those who write best code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer in your ability established. So up coming time you squash a bug, have a instant to reflect—you’ll appear away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and persistence — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to become superior at Everything you do.